WordPress, being one of the most popular Content Management Systems (CMS) worldwide, powers millions of websites. However, its widespread use also makes it a prime target for malicious actors seeking to exploit potential vulnerabilities. As a website owner, WordPress developer, website maintainer, or administrator, safeguarding your WordPress site from security threats is more important today than ever.
Note this article pertains to writing PHP code inside WordPress Theme or Plugin template files. Escaping is not something used in the WordPress page editor. Understanding this concept is important to ensure your website designer or web developer follows best practices.
Enhancing WordPress Security by Escaping Content
This article will explore the significance of appropriately escaping HTML content in custom WordPress theme template files to improve security. We will address the potential hazards of not escaping HTML content and emphasize why it is crucial to apply escaping methods. We will also explain how to implement these techniques to safeguard your website's security.
Additionally, we'll provide in-depth code examples to demonstrate the usage of the esc_html()
and wp_kses_post()
functions for securing advanced custom fields field output and core fields such as the_title()
.
What is Code Escaping?

Escaping, sanitization, and validation are crucial practices in programming to protect applications from various security threats and WordPress' common vulnerabilities. It involves processing and validating user-provided data to ensure it is safe and free from potentially harmful content, such as malicious code or injection attacks.
The process of code security escaping involves identifying and neutralizing special characters, HTML tags, SQL queries, or other harmful inputs that could lead to code execution, data breaches, or other security vulnerabilities. By escaping or sanitizing user inputs, developers can prevent potential exploits, like Cross-Site Scripting (XSS) attacks, SQL injection,
Understanding Escaping in WordPress
Before we delve into the specifics of escaping HTML content, let's take a moment to understand the concept of escaping and its significance in WordPress security.
Escaping is the process of converting special characters and HTML tags into their corresponding HTML entities, ensuring they are rendered safely on the front end. This process prevents malicious code from being executed and displayed as-is, thus mitigating various security threats.
By default, WordPress automatically escapes most user-generated content before rendering it on the front end, which provides a certain level of protection. However, there are instances where developers must manually apply escaping to avoid potential security vulnerabilities.
It is crucial to implement escaping to prevent accidental damage to the markup of a website and also for security reasons. When a user enters quotes or symbols through your plugin, it can potentially disrupt the markup, so it is essential to escape such output. However, it is important to exercise caution while sanitizing input since some characters may be necessary for legitimate purposes. See the WordPress VIP Guidelines for Escaping
Guiding Principles for Escaping in WordPress
Source: WordPress VIP
- Never trust user input.
- Sanitation is okay, but validation/rejection is better.
- Validation on the client-side is for the user’s benefit; validation/sanitization on the server-side is always needed.
- Never assume anything.
- Escape everything from untrusted sources (e.g., databases and users), third-parties (e.g., Twitter), etc.
- Escape as late as possible.
The Security Implications of Unescaped HTML Content

When users have the ability to input HTML content into fields, such as the title field, there is a risk of security breaches. Administrators can easily enter arbitrary HTML content into these fields, which opens up potential attack vectors.
Let's explore some of the security risks associated with unescaped HTML content:
1. Rogue Administrator Adds Malicious JavaScript
A malicious administrator accessing the title field via editing or adding a post or page title can deliberately inject a <script>
tag containing harmful JavaScript code. When the title is displayed on the front end, the injected script will execute, potentially compromising users' data or spreading malware.
2. Hacker Exploits and Changes Data
If a hacker successfully exploits a vulnerability on your website, they may gain unauthorized access to your administrative features and manipulate the title field content. This could lead to malicious code injection, defacing your site, or unauthorized access to sensitive information.
3. Compromised Plugin Manipulates Data
If a plugin on your website is compromised, the attacker who has gained access to your server could use a filter to alter the content and insert malicious code into the plugin. Since plugins have extensive access to the site's functionalities, a compromised plugin poses a significant threat to your website's security.
4. Broken Plugin Allows Content Changes
If a plugin is not properly implemented or has a flaw, it may permit users to modify content in the database. In the event that this occurs, it could enable malevolent individuals to inject harmful content into the title, which could lead to the website's integrity and reputation being compromised. Therefore, it's critical to ensure that any plugins utilized are correctly installed and functioning as intended to prevent such incidents from occurring.
5. Hacker Modifies Cached Content
Hackers who gain unauthorized access to caching systems like Redis, APC, or Memcached could manipulate cached content to include malicious scripts or links. As a result, all users accessing the cached version of the title will be exposed to the malicious content.
6. File-Based Cache Compromise
File-based caching mechanisms are also susceptible to attacks, where attackers can tamper with cached data and insert harmful content. This poses a significant risk as cached content is often served to users without direct interaction with the website's backend.
Mitigate WordPress Security Risks by Escaping Content

To combat these potential security vulnerabilities, escaping becomes an indispensable practice. Proper escaping ensures that any user-generated content displayed on the front end is rendered safely and as intended, eliminating the risk of harmful content being executed or displayed unintendedly.
Escaping not only prevents the execution of malicious scripts but also safeguards your website's integrity by maintaining the original content intended by the user. By following WordPress's recommended escaping functions, developers can enhance their website's overall security posture.
Let's explore two key WordPress functions used for escaping HTML content: esc_html()
and wp_kses_post()
. We will learn how to implement these functions to secure the title field effectively.
Using esc_html()
for Safe Text Output
The esc_html()
function is an essential tool for rendering HTML content safely on your WordPress site. When used, this function escapes any HTML tags and special characters in the provided string, ensuring it is safe for output.
We will use the Post Title; the_title()
in our example to make it simple to demonstrate.
You should use the esc_html()
function to display the title when outputting the title content safely. This way, even if the title contains HTML tags or special characters, they will be converted to their corresponding entities and displayed harmlessly.
Here's how you can implement esc_html()
to secure the title field:
PHP<?php echo esc_html(get_the_title()); ?>
PHP
By using esc_html(),
You are guaranteed that any user-entered HTML content is rendered safely and without risking security breaches. This function is particularly useful when displaying the title content without allowing any HTML tags.
Using wp_kses_post()
for Including Allowed HTML Tags
In certain cases, you may want to allow specific HTML tags in the title field while ensuring they are safe for display. The wp_kses_post()
function comes in handy in such scenarios. This function sanitizes the content, allowing only the specified tags and attributes while escaping any disallowed tags.
To utilize wp_kses_post() and allow specific HTML tags in the title field, follow this code example:
PHP<?php echo wp_kses_post(get_the_content()); ?>
PHP
With wp_kses_post()
, you maintain control over which HTML tags are allowed in the title field, reducing the risk of potential security breaches while still permitting certain tags for formatting or stylistic purposes.
Escaping Custom Field Output
When using Advanced Custom Fields (ACF) in custom WordPress theme template files, it's essential to escape or sanitize the output to prevent security vulnerabilities and ensure data integrity. Here are some examples of how you can do it:
- Escaping for HTML output using '
esc_html
' to escape text output - Escaping for HTML Attributes output using '
esc_attr
' to escape attribute output - Escaping for Rich Text output using '
wp_kses_post
' to escape HTML output while allowing some safe tags - Escaping for URL output using '
esc_url
' to escape URL output and 'esc_url_raw
' to escape URLs without any additional encoding - Escaping for JavaScript output using.'
esc_js
' to escape JavaScript output - Sanitizing for CSS output using '
sanitize_text_field
' to sanitize CSS output
Code Examples below for escaping ACF ( Advanced Custom Fields) data:
PHP<?php
PHP
// Using 'esc_html' to escape text output
echo esc_html( get_field( 'field_name' ) );
// Using 'esc_html' to escape text output with a php variable set first
$field_name_variable = get_field( 'field_name' );
echo esc_html( $field_name_variable) );
// Using 'esc_attr' to escape attribute output
echo '<div class="' . esc_attr( get_field( 'field_name' ) ) . '">Content</div>';
// Using 'wp_kses_post' to escape HTML output while allowing some safe tags
echo wp_kses_post( get_field( 'field_name' ) );
// Using 'esc_url' to escape URL output
echo esc_url( get_field( 'field_name' ) );
// Using 'esc_url_raw' to escape URLs without any additional encoding
echo esc_url_raw( get_field( 'field_name' ) );
// Using 'esc_js' to escape JavaScript output
echo '<script> var data = ' . esc_js( json_encode( get_field( 'field_name' ) ) ) . '; </script>';
// Using 'sanitize_text_field' to sanitize CSS output
echo '<style> .class-name { property: ' . sanitize_text_field( get_field( 'field_name' ) ) . '; } </style>';
?>
These examples demonstrate different escaping and sanitization functions available in WordPress to ensure the safe output of data retrieved from ACF fields in custom template files. Always choose the appropriate function based on the output context to ensure proper security measures are in place.
Enhancing the security of your WordPress website through escaping content output
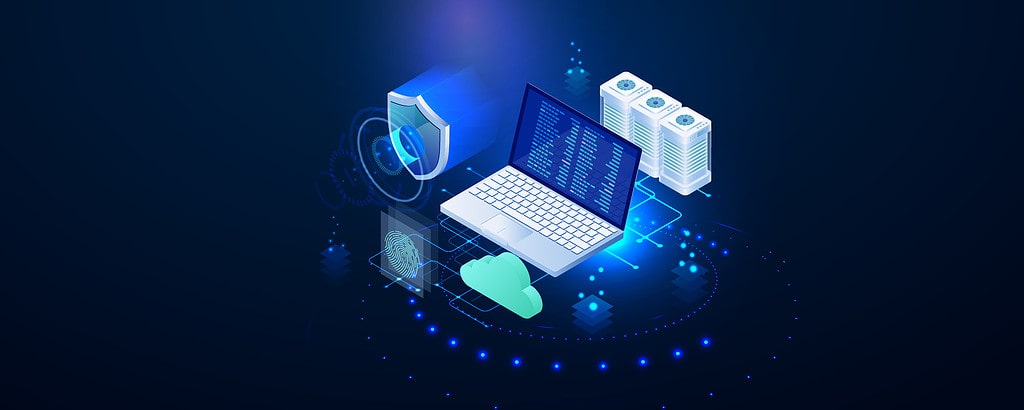
Enhancing the security of your WordPress website should be an ongoing and top-priority endeavor. With the vast amount of user-generated content and potential attack vectors, mitigating security risks is crucial to safeguarding your website and user data.
Escaping HTML content in WordPress templates is an indispensable security practice that ensures user-generated content is displayed safely on the front end. By employing WordPress's core escaping functions, esc_html()
and wp_kses_post()
, you can confidently render HTML content without risking security vulnerabilities.
Remember, escaping is just one aspect of a robust security strategy. It should always complement other security practices, such as data validation, input sanitization, and keeping your WordPress installation, plugins, and themes updated to their latest secure versions.
Adhering to best practices and proactively addressing security concerns can create a safer online environment for website administrators and visitors.
Keeping your website up to date is one of the most important security decisions you can make for your website. We provide complete website maintenance hosting and support to assist your organization's website security goals.
Additional Resources and Guides for Escaping in WordPress
- WordPress VIP Guidelines for Escaping
- Sanitizing Data in WordPress
- Escaping Data in WordPress
- Validating Data in WordPress
- Common Vulnerabilities in WordPress
- Sanitizing, Escaping, and Encoding
Escaping in WordPress FAQs
What is the escape function in WordPress?
It is important to utilize techniques such as Escaping when considering security measures. This particular technique can assist in safeguarding your data by providing context before it is presented to the end user. To guarantee that the output is secure, it is recommended to utilize the sanitize_*() WordPress helper functions that are readily available.
What is the difference between escaping and sanitizing?
The security industry seems to have adopted the term "escaping" as a replacement for "encoding." This new term describes the process of converting special characters into a reversible code. In contrast, sanitization involves the permanent deletion of special characters.
How do I use the esc_html()
function in WordPress?
You should use the function to safely display the title content, even if it contains HTML tags or special characters. This way, they will be converted to their corresponding entities and displayed without harm.
Here's how you can implement esc_html()
to secure the title field:
<?php echo esc_html(get_the_title()); ?>